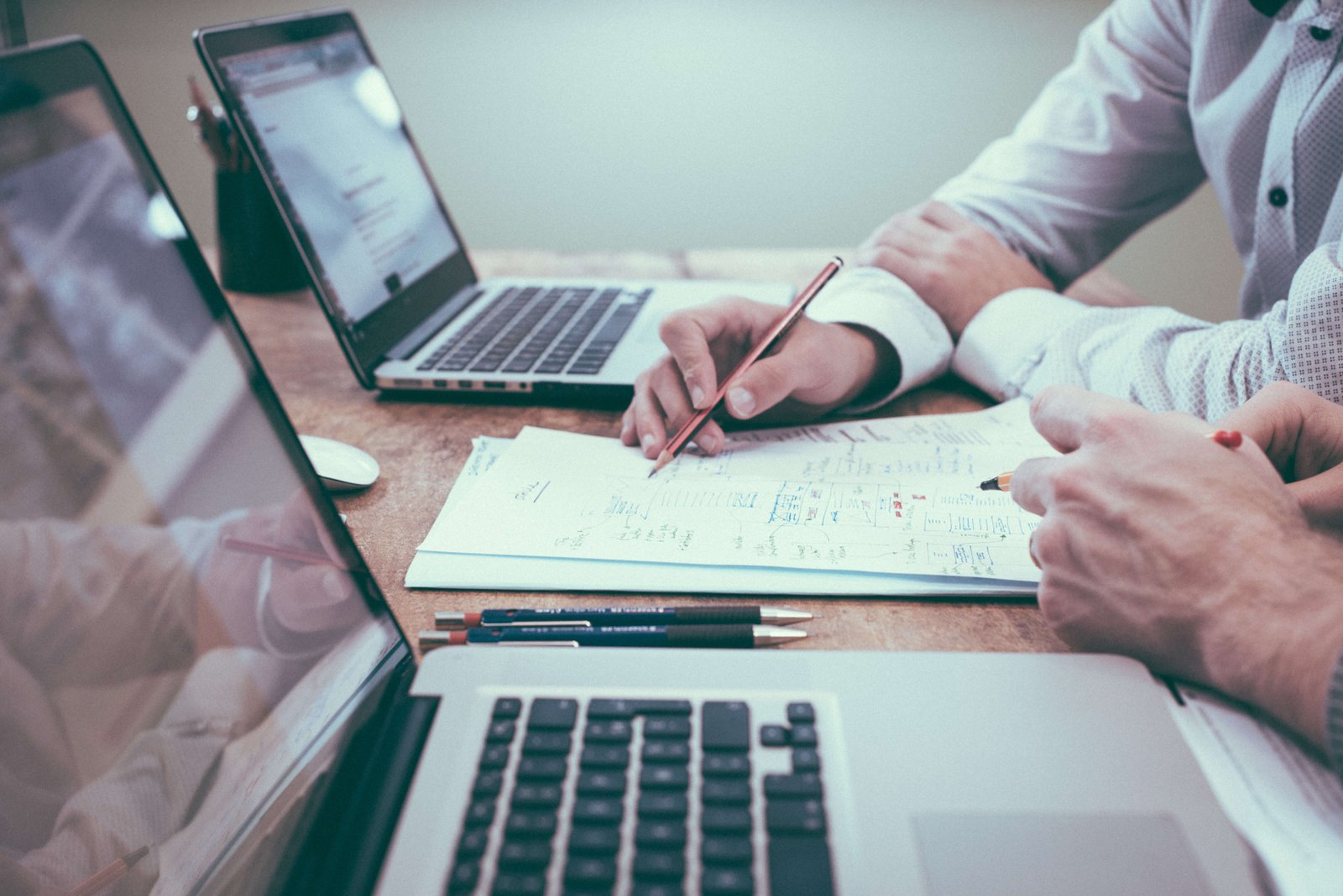
Creational Design Patterns in System Development
By Marcelo Vieyra, October 30th 2023 | 3 mins, 425 words
Introduction to Creational Design Patterns
Creational design patterns focus on how to create objects and object structures in a system. These patterns address common design problems such as the need to create objects in various circumstances or the efficient creation of complex objects. Some of the most well-known creational patterns include:
1. Singleton Pattern
The Singleton pattern ensures that a class has a single instance and provides a global point of access to that instance. This is useful when only one instance of a class is needed to coordinate actions throughout the system. Here's an example in PHP:
class Singleton { private static $instance; private function __construct() { } public static function getInstance() { if (self::$instance === null) { self::$instance = new Singleton(); } return self::$instance; } }
2. Factory Method Pattern
The Factory Method pattern defines an interface for creating objects but allows subclasses to alter the type of objects to be created. This promotes object creation based on inheritance. Here's an example in PHP:
abstract class Creator { abstract public function factoryMethod(); } class ConcreteCreatorA extends Creator { public function factoryMethod() { return new ConcreteProductA(); } }
3. Abstract Factory Pattern
The Abstract Factory pattern provides an interface for creating families of related objects without specifying their concrete classes. This is useful to ensure that the created objects are consistent in their design. Here's an example in PHP:
interface AbstractFactory { public function createProduct1(); public function createProduct2(); } class ConcreteFactory1 implements AbstractFactory { public function createProduct1() { return new Product1A(); } public function createProduct2() { return new Product2A(); } }
4. Builder Pattern
The Builder pattern separates the construction of a complex object from its representation, allowing different ways to build objects. This is useful when you want to create objects with custom configurations. Here's an example in PHP:
class Product { // Complex product class } interface Builder { public function buildPart1(); public function buildPart2(); public function getResult(); } class ConcreteBuilder implements Builder { private $product; public function buildPart1() { // Logic to build part 1 } public function buildPart2() { // Logic to build part 2 } public function getResult() { return $this->product; } }
5. Prototype Pattern
The Prototype pattern is used to create new objects by copying an existing object, known as the prototype. This is useful when object creation is costly or complicated. Here's an example in PHP:
class Prototype { private $property; public function __construct() { $this->property = 1; } public function clone() { $clone = new self(); $clone->property = $this->property; return $clone; } }
Conclusion
Creational design patterns are essential tools in software system development. They help ensure that object creation is flexible, decoupled, and efficient, which, in turn, facilitates system maintenance and scalability. By understanding and applying these patterns, developers can optimize object creation in their projects and build more robust and easily maintainable systems.